|
简述 在spingMVC框架中,所有的类都是通过springMVC容器来加载和实例化的。
因此在junit测试的时候可以在其初始化方法中去加载spring配置文件。所有用Junit进行单元测试,都需要下面的配置。注:配置文件的加载根据自己项目的实际情况进行配置。
@RunWith(SpringJUnit4ClassRunner.class)
@WebAppConfiguration
@ContextConfiguration(locations = { "classpath:config/spring-mvc.xml",
"classpath:config/spring-resources.xml",
"classpath:config/spring-application.xml",
"classpath:config/spring-security.xml" }) |
Dao层的单元测试 通过依赖注入的方式注入测试对象;
package com.lczyfz.zerobdt.test;
import com.lczyfz.zerobdt.modules.test.dao.TestDao;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import org.springframework.transaction.annotation.Transactional;
import static junit.framework.TestCase.assertEquals;
/**
* Created by maple on 2018-07-01.
*/
@RunWith(SpringJUnit4ClassRunner.class)
@Transactional
@ContextConfiguration(locations = {"/spring-context.xml"})
public class TestDaoTests {
@Autowired
TestDao testDao;
@Test
public void testFindAllList(){
System.out.println(testDao.findAllList(new com.lczyfz.zerobdt.modules.test.entity.Test()).toString());
assertEquals(testDao.findAllList(new com.lczyfz.zerobdt.modules.test.entity.Test()).size(),13);
}
} |
成功:
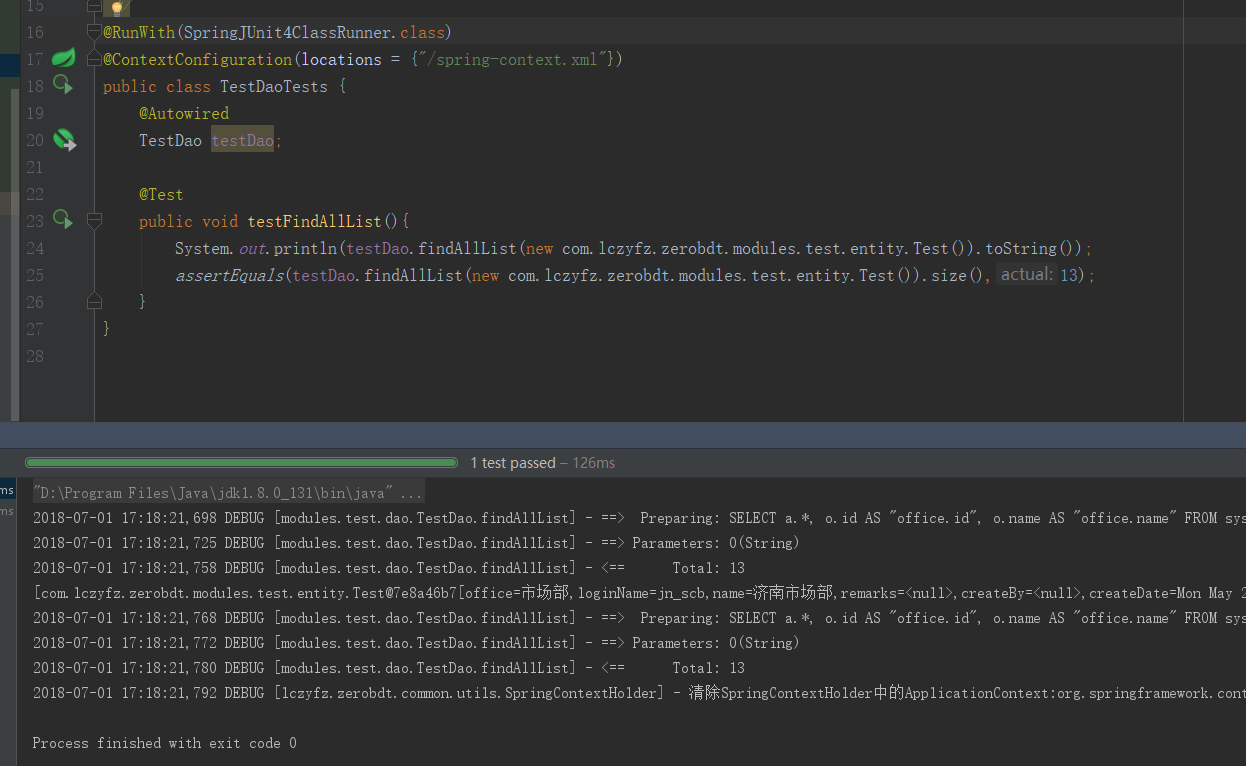
失败:
Service层的单元测试 和Dao层类似;
package com.lczyfz.zerobdt.test;
import com.lczyfz.zerobdt.modules.test.service.TestService;
import junit.framework.TestCase;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import static junit.framework.TestCase.assertEquals;
import static org.junit.Assert.*;
/**
* Created by maple on 2018-07-01.
*/
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(locations = {"/spring-context.xml"})
public class TestDaoServices {
@Autowired
TestService testService;
@Test
public void testFindAllList(){
System.out.println(testService.get("5").toString());
assertNotNull(testService.get("5"));
}
} |
Controller层的单元测试 package com.lczyfz.zerobdt.test;
import com.lczyfz.zerobdt.common.test.SpringTransactionalContextTests;
import com.lczyfz.zerobdt.modules.test.web.TestController;
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import org.springframework.test.web.servlet.MockMvc;
import org.springframework.test.web.servlet.MvcResult;
import org.springframework.test.web.servlet.ResultActions;
import org.springframework.test.web.servlet.request.MockMvcRequestBuilders;
import org.springframework.test.web.servlet.setup.MockMvcBuilders;
import java.io.UnsupportedEncodingException;
import static org.junit.Assert.assertNotNull;
/**
* Created by maple on 2018-07-01.
*/
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(locations = {"/spring-context.xml","/spring-mvc.xml"})
public class TestDaoControllers extends SpringTransactionalContextTests{
@Autowired
TestController testController;
MockMvc mockMvc;
@Before
public void setup(){
mockMvc = MockMvcBuilders.standaloneSetup(testController).build();
}
@Test
public void testFindAllList(){
ResultActions resultActions = null;
try {
resultActions = this.mockMvc.perform(MockMvcRequestBuilders.post("/show_user").param("id", "1"));
} catch (Exception e) {
e.printStackTrace();
}
MvcResult mvcResult = resultActions.andReturn();
String result = null;
try {
result = mvcResult.getResponse().getContentAsString();
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
System.out.println("=====客户端获得反馈数据:" + result);
// 也可以从response里面取状态码,header,cookies...
// System.out.println(mvcResult.getResponse().getStatus());
}
} |
Controller层的测试建议采用Postman的方式来测试,可以记录url,同时也更符合实际情况。
----------------------------
原文链接:https://www.jianshu.com/p/8d0f7201b445
程序猿的技术大观园:www.javathinker.net
[这个贴子最后由 flybird 在 2020-11-24 20:26:14 重新编辑]
|
|