|
本文参考《精通JPA与Hibernate:Java对象持久化技术详解》,作者:孙卫琴,清华大学出版社出版
Hibernate API和JPA API中主要接口之间有一些对应关系,例如:
JPA API ----------------------- Hibernate API
EntityManagerFactory ----- SessionFactory
EntityManager ---------------- Session
EntityTransaction ------------- Transaction
SessionFactory接口在JPA API中的对等接口是javax.persistence.EntityManagerFactory;Session接口在JPA API中的对等接口是javax.persistence.EntityManager;Transaction接口在JPA API中的对等接口是javax.persistence.EntityTransaction。Query接口在JPA API中的对等接口是javax.persistence.Query。
EntityManager接口提供了操纵数据库的各种方法,如:
(1) persist()方法:把Java对象保存到数据库中。等价于Session接口的persist()方法。
(2) merge()方法:保存或更新数据库中的Java对象。等价于Session接口的merge()方法。
(3) remove()方法:把特定的Java对象从数据库中删除。类似于Session接口的delete()方法。EntityManager接口的remove()方法只能删除持久化状态的对象,而Session接口的delete()方法可以删除持久化状态或游离状态的对象。。
(4) find()方法:从数据库中加载Java对象。等价于Session接口的get()方法。
下面这个BusinessService类通过JPA API来访问数据库:package mypack;
import javax.persistence.EntityManager;
import javax.persistence.EntityManagerFactory;
import javax.persistence.Persistence;
import javax.persistence.EntityTransaction;
import javax.persistence.Query;
import java.io.*;
import java.sql.Date;
import java.sql.Timestamp;
import java.util.*;
public class BusinessService{
public static EntityManagerFactory entityManagerFactory;
/** 初始化JPA,创建EntityManagerFactory实例 */
static{
try{
entityManagerFactory=
Persistence.createEntityManagerFactory( "myunit" );
}catch(Exception e){
e.printStackTrace();
throw e;
}
}
/** 查询所有的Customer对象,
然后调用printCustomer()方法打印Customer对象信息 */
public void findAllCustomers(PrintWriter out)throws Exception{
EntityManager entityManager =
entityManagerFactory.createEntityManager();
EntityTransaction tx = null;
try {
tx = entityManager.getTransaction();
tx.begin(); //开始一个事务
Query query=entityManager.createQuery(
"from Customer as c order by c.name asc");
List customers=query.getResultList();
for (Iterator it = customers.iterator(); it.hasNext();) {
printCustomer(out,(Customer) it.next());
}
tx.commit(); //提交事务
}catch (RuntimeException e) {
if (tx != null) {
tx.rollback();
}
throw e;
} finally {
entityManager.close();
}
}
/** 持久化一个Customer对象 */
public void saveCustomer(Customer customer){
EntityManager entityManager =
entityManagerFactory.createEntityManager();
EntityTransaction tx = null;
try {
tx = entityManager.getTransaction();
tx.begin();
entityManager.persist(customer);
tx.commit();
}catch (RuntimeException e) {
if (tx != null) {
tx.rollback();
}
throw e;
} finally {
entityManager.close();
}
}
/** 按照OID加载一个Customer对象,然后修改它的属性 */
public void loadAndUpdateCustomer(Long customer_id,String address){
EntityManager entityManager =
entityManagerFactory.createEntityManager();
EntityTransaction tx = null;
try {
tx = entityManager.getTransaction();
tx.begin();
Customer c=entityManager
.find(Customer.class,customer_id);
c.setAddress(address);
tx.commit();
}catch (RuntimeException e) {
if (tx != null) {
tx.rollback();
}
throw e;
} finally {
entityManager.close();
}
}
/**删除Customer对象 */
public void deleteCustomer(Customer customer){
EntityManager entityManager =
entityManagerFactory.createEntityManager();
EntityTransaction tx = null;
try {
tx = entityManager.getTransaction();
tx.begin();
//获得持久化状态的Customer对象
Customer c=entityManager
.find(Customer.class,customer.getId());
entityManager.remove(c);
tx.commit();
}catch (RuntimeException e) {
if (tx != null) {
tx.rollback();
}
throw e;
} finally {
entityManager.close();
}
}
/** 把Customer对象的信息输出到控制台,如DOS 控制台*/
private void printCustomer(PrintWriter out,Customer customer)
throws Exception{……}
public void test(PrintWriter out) throws Exception{……}
public static void main(String args[]) throws Exception{
new BusinessService2().test(new PrintWriter(System.out,true));
entityManagerFactory.close();
}
} |
对JPA的初始化非常简单,只要通过javax.persistence.Persistence的静态方法createEntityManagerFactory()来创建EntityManagerFactory对象:
entityManagerFactory=
Persistence.createEntityManagerFactory( "myunit" ); |
以上Persistence.createEntityManagerFactory( “myunit” )方法中的参数“myunit”指定持久化单元包的名字。JPA会到persistence.xml配置文件中读取相应的持久化单元包中的配置信息。
所有访问数据库的操作都使用以下流程:
EntityManager entityManager =
entityManagerFactory.createEntityManager();
EntityTransaction tx = null;
try {
tx = entityManager.getTransaction();
tx.begin(); //声明开始事务
//执行查询、保存、更新和删除等各种数据访问操作
……
tx.commit(); //提交事务
}catch (RuntimeException e) {
if (tx != null)
tx.rollback();
throw e;
} finally {
entityManager.close();
} |
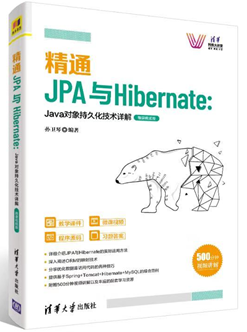
程序猿的技术大观园:www.javathinker.net
[这个贴子最后由 admin 在 2021-10-09 10:44:02 重新编辑]
|
|